Leetcode – 8. String to Integer (atoi)
Link: https://leetcode.com/problems/string-to-integer-atoi/description/
Problem
Implement the myAtoi(string s)
function, which converts a string to a 32-bit signed integer.
The algorithm for myAtoi(string s)
is as follows:
- Whitespace: Ignore any leading whitespace (
" "
). - Signedness: Determine the sign by checking if the next character is
'-'
or'+'
, assuming positivity if neither present. - Conversion: Read the integer by skipping leading zeros until a non-digit character is encountered or the end of the string is reached. If no digits were read, then the result is 0.
- Rounding: If the integer is out of the 32-bit signed integer range
[-231, 231 - 1]
, then round the integer to remain in the range. Specifically, integers less than-231
should be rounded to-231
, and integers greater than231 - 1
should be rounded to231 - 1
.
Return the integer as the final result.
Solution
Idea
My idea is straightforward. We just read character in the string one by one. If we have not encounter a number, there’re some special cases we need to handle:
– leading space/zero.
– positive/negative sign. also this sign will start a number.
Next we will check the character is number or not. If it’s a number, add it to the result string. If not, break the loop.
Before return the result, we need to handle the rounding then it’s good.
Code
/**
* @param {string} s
* @return {number}
*/
var myAtoi = function(s) {
let numberStart = false;
let result = '0';
let positive = 1;
for (let i = 0; i < s.length; i++) {
const char = s[i];
// if we have not encounter a number, we can skip leading space
// also can check "-", "+" for negative/positive number
if (!numberStart) {
if (char === ' ' || char === '0') continue;
if (char === '-') {
numberStart = true;
positive = -1;
continue;
}
if (char === '+') {
numberStart = true;
continue;
}
}
if (isCharNumber(char)) {
numberStart = true;
result = result.toString() + char;
} else { // not a number --> break
break;
}
}
result = parseInt(result) * positive;
if (result < -2147483648) return -2147483648;
if (result > 2147483647) return 2147483647;
return result;
};
function isCharNumber(c) {
return c >= '0' && c <= '9';
}
Result
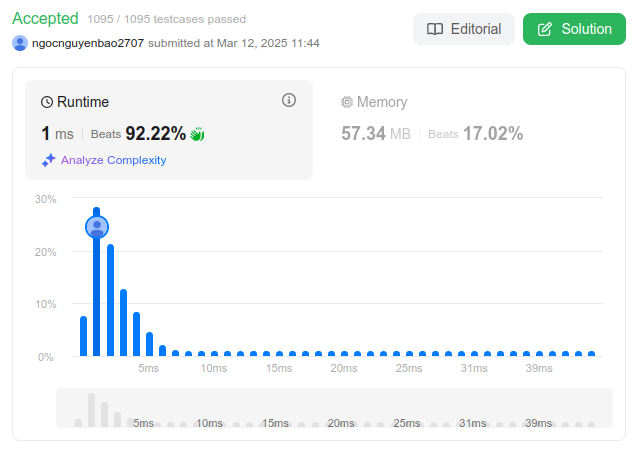