Leetcode – 9. Palindrome Number (Easy)
Link: Palindrome Number – LeetCode
Problem
Given an integer x
, return true
if x
is a palindrome, and false
otherwise.
Example 1:
Input: x = 121 Output: true Explanation: 121 reads as 121 from left to right and from right to left. Example 2:
Input: x = -121 Output: false Explanation: From left to right, it reads -121. From right to left, it becomes 121-. Therefore it is not a palindrome. Example 3:
Input: x = 10
Output: false
Explanation: Reads 01 from right to left. Therefore it is not a palindrome.
Solution
Idea
This is an easy problem. My idea is:
– convert x to string
– loop from the start to the middle of the string
– compare the character from the start and end of the string
Code
/**
* @param {number} x
* @return {boolean}
*/
var isPalindrome = function(x) {
let str = x.toString();
let count = Math.floor(str.length / 2);
let result = true;
for (let i = 0; i < count; i++) {
if (str[i] === str[str.length - i - 1]) {
continue;
}
result = false;
break;
}
return result;
};
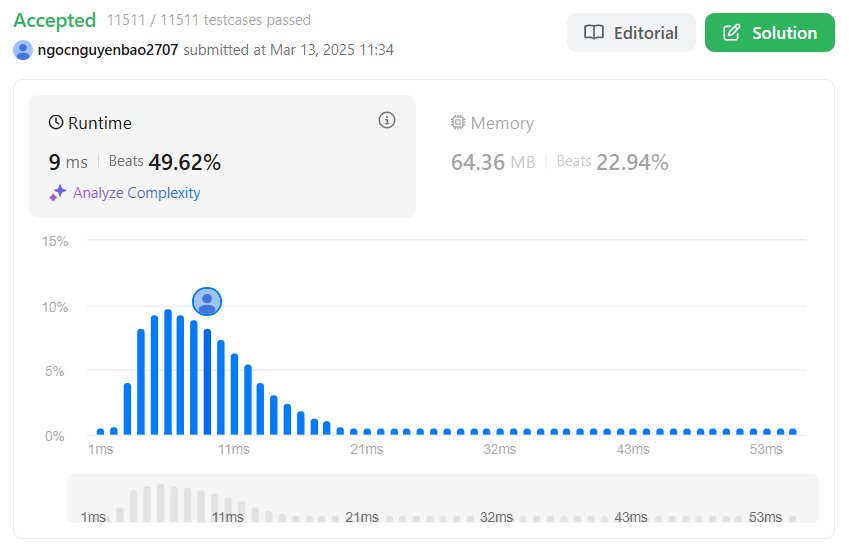