Leetcode – 12. Integer to Roman
Problems
Example 1:
Input: num = 3749
Output: “MMMDCCXLIX”
Explanation:
3000 = MMM as 1000 (M) + 1000 (M) + 1000 (M) 700 = DCC as 500 (D) + 100 (C) + 100 (C) 40 = XL as 10 (X) less of 50 (L) 9 = IX as 1 (I) less of 10 (X) Note: 49 is not 1 (I) less of 50 (L) because the conversion is based on decimal places
Example 2:
Input: num = 58
Output: “LVIII”
Explanation:
50 = L 8 = VIII
Example 3:
Input: num = 1994
Output: “MCMXCIV”
Explanation:
1000 = M
900 = CM
90 = XC
4 = IV
Solution
Idea
I spent quite a lot of time thinking about the solution before coding. It’s off because we all know the brute force way by mapping all the possible number to roman characters and do it.
I found a way to retrieve 1 digit at a time and calculate the appropriate roman characters for it. I still need to handle the 4 and 9 cases by themselves. The result is quite good.
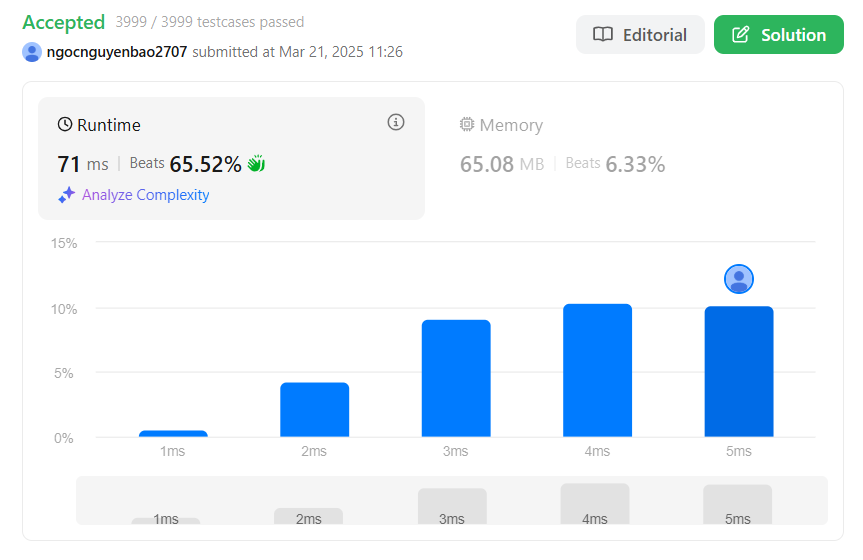
Code
/**
* @param {number} num
* @return {string}
*/
var intToRoman = function(num) {
let romans = {
1: 'I',
5: 'V',
10: 'X',
50: 'L',
100: 'C',
500: 'D',
1000: 'M'
}
let power = 3;
let result = '';
while (power >= 0) {
let decimal = 10 ** power;
let digit = Math.floor((num / decimal) % 10)
if (digit === 4) {
result += romans[decimal] + romans[decimal * 5];
power--;
continue;
}
if (digit === 9) {
result += romans[decimal] + romans[decimal * 10];
power--;
continue;
}
if (digit >= 5) {
result += romans[decimal * 5];
digit -= 5;
}
while (digit > 0) {
digit -= 1;
result += romans[decimal];
}
power--;
}
return result;
};