Leetcode – 16. 3sum closest (yeah… threesum again)
Problems
Given an integer array nums
of length n
and an integer target
, find three integers in nums
such that the sum is closest to target
. Return the sum of the three integers.
You may assume that each input would have exactly one solution.
Solution
After solving the 3sum problem, I think that this problem is pretty easy. We will use two pointer technique to solve it.
1. sort the array of integers
2. loop through the array with i start from 0, left = i + 1, right = length – 1.
3. check the sum of the three numbers
– sum > target –> reduce the right
– sum < target –> increase the left
– get the difference between sum and target and store it if it’s the closest
– if the difference is 0, break the loop and return.
/**
* @param {number[]} nums
* @param {number} target
* @return {number}
*/
var threeSumClosest = function(nums, target) {
let diff = 999999;
let result = undefined;
nums.sort((a, b) => a - b);
for (let i = 0; i < nums.length - 2; i++) {
// ignore duplicated cases
if (i >= 1 && nums[i] === nums[i - 1]) continue;
let l = i + 1;
let r = nums.length - 1;
while (l < r) {
const sum = nums[i] + nums[l] + nums[r];
let d = Math.abs(sum - target);
if (d < diff) {
diff = d;
result = sum;
}
if (sum > target) {
r--;
} else if (sum < target) {
l++;
} else {
break;
}
}
if (diff === 0) {
break;
}
}
return result;
};
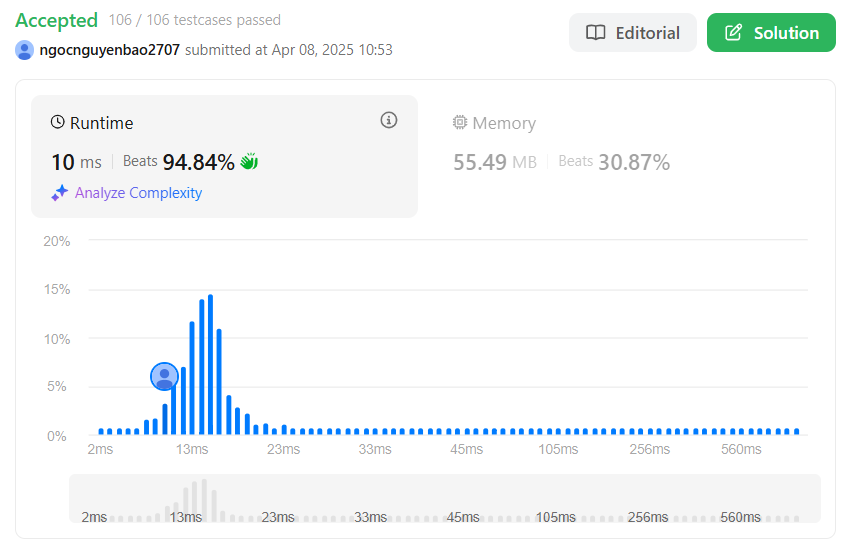