Ignore files and folders to sync from Dropbox
Contents
What is it?
I have just purchased Dropbox premium account and I want to try to move all of my code to Dropbox so that I can I have it on my other pc/laptop. However, I want to ignore some files/folders such as node_modules, vendor, package-lock.json… because they’re heavy and may be different on each device.
How to do it?
Windows
First we need to understand how to ignore file with Dropbox. Read the link here: How to set a file or folder to be ignored – Dropbox Help.
Unfortunately, Dropbox does not provide a solution with UI or something convenient to do. We need to run command and ignore file by file, folder by folder. Example command in Windows Powershell:
Set-Content -Path 'C:\Users\yourname\Dropbox(Personal)\YourFileName.pdf' -Stream com.dropbox.ignored -Value 1
Luckily, I found a guy on Stackoverflow who posted a genius solution: node.js – How to ignore node_modules recursively in Dropbox on a Mac – Stack Overflow. However, it’s for mac, not windows. I used an online tool to convert it to Powershell script.
Linux
attr -s com.dropbox.ignored -V 1 /Users/yourname/Dropbox\ \(Personal\)/YourFileName.pdf
Final result
Windows
I created a ps1 file (powershell script file) and here’s the final code:
# ignore node_modules and vendor folder
Get-ChildItem -Path D:\Dropbox -Directory -Recurse | Where-Object {
($_.FullName -like '*node_modules' -and $_.FullName -notmatch '\\node_modules\\' -and $_.FullName -notmatch '\\vendor\\') -or ($_.FullName -like '*vendor' -and $_.FullName -notmatch '\\vendor\\' -and $_.FullName -notmatch '\\node_modules\\' -and $_.FullName -notmatch '\\js\\vendor' -and $_.FullName -notmatch '\\public\\vendor' -and $_.FullName -notmatch '\\views\\vendor' -and $_.FullName -notmatch '\\wsdltophp\\' )
} | ForEach-Object {
$command = "Set-Content -Path ""$($_.FullName)"" -Stream com.dropbox.ignored -Value 1"
Write-Output $command
Invoke-Expression $command
}
# ignore package-lock.json, composer.lock, yarn.lock and .env file
Get-ChildItem -Path D:\Dropbox -File -Recurse | Where-Object { ($_.FullName -like '*package-lock.json' -or $_.FullName -like '*composer.lock' -or $_.FullName -like '*yarn.lock' -or $_.FullName -like '*.env') -and $_.FullName -notmatch '\\node_modules\\' -and $_.FullName -notmatch '\\vendor\\' -and $_.FullName -notmatch '\\wsdltophp\\' } | ForEach-Object {
$command = "Set-Content -Path ""$($_.FullName)"" -Stream com.dropbox.ignored -Value 1"
Write-Output $command
Invoke-Expression $command
}
The script run beautifully. Now every time I add new project to Dropbox, I just need to rerun the script file.
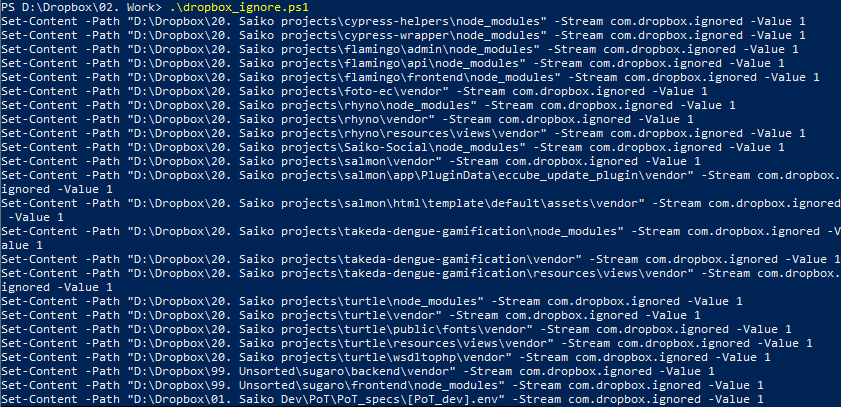
Linux
#!/bin/bash
# ignore node_modules and vendor folder
find ~/Dropbox -type d \( -name "node_modules" -and ! -path "*/node_modules/*" -and ! -path "*/vendor/*" \) -o \( -name "vendor" -and ! -path "*/vendor/*" -and ! -path "*/node_modules/*" -and ! -path "*/js/vendor/*" -and ! -path "*/public/vendor*" -and ! -path "*/views/vendor*" -and ! -path "*/wsdltophp/*" -and ! -path "*/eccube_update_plugin/*" -and ! -path "*/assets/vendor*" -and ! -path "*/assets/js/vendor*" \) | while read -r dir; do
command="attr -s com.dropbox.ignored -V 1 \"$dir\""
echo "$command"
eval "$command"
done
# ignore package-lock.json, composer.lock, yarn.lock and .env file
find ~/Dropbox -type f \( -name "package-lock.json" -o -name "composer.lock" -o -name "yarn.lock" -o -name ".env" \) -a ! -path "*/node_modules/*" -a ! -path "*/vendor/*" -a ! -path "*/wsdltophp/*" -a ! -path "*/eccube_update_plugin/*" | while read -r file; do
command="attr -s com.dropbox.ignored -V 1 \"$file\""
echo "$command"
eval "$command"
done
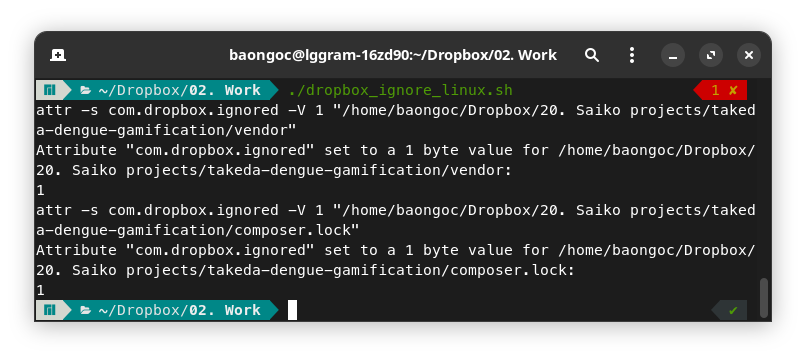