Leetcode – 14. Longest common prefix
Problem
Write a function to find the longest common prefix string amongst an array of strings.
If there is no common prefix, return an empty stringĀ ""
.
Solution
First version
This is an easy problem. My idea is:
– Loop through the first string in the array.
– Loop through other strings in the array and compare the characters in the same position.
– if the characters are not matched –> break through the loop.
– if the characters are matched, add it to the result.
/**
* @param {string[]} strs
* @return {string}
*/
var longestCommonPrefix = function(strs) {
let result = "";
let baseStr = strs[0];
if (strs.length === 1) return baseStr;
for (let i = 0; i < baseStr.length; i++) {
let matched = true;
for (let j = 1; j < strs.length; j++) {
if (strs[j][i] !== baseStr[i]) {
matched = false;
break;
}
}
if (matched) {
result += baseStr[i];
} else {
break;
}
}
return result;
};
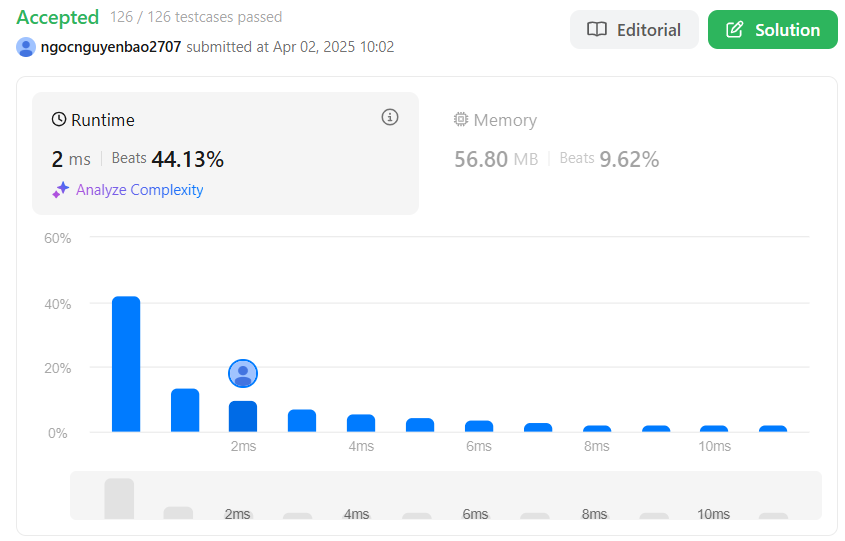
Second version
To improve the speed, we need to reduce the number of loop. Instead of comparing the string one by one, if we sort them first, we only need to compare the first and the last string because they will be the most different.
const solution2 = function(strs) {
strs = strs.sort();
let result = "";
let first = strs[0];
let last = strs[strs.length - 1];
for (let i = 0; i < first.length; i++) {
if (first[i] === last[i]) {
result += first[i];
} else {
break;
}
}
return result;
}
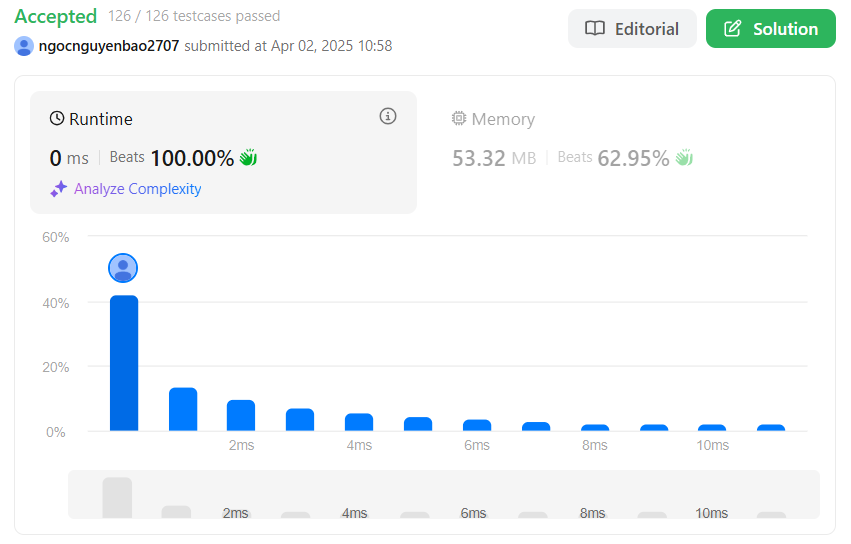